Pip install
is the command you use to install Python packages with the Pip package manager. If you’re wondering what Pip stands for, the name Pip is a recursive acronym for ‘Pip installs packages’. There are two ways to
install Python packages with pip:
- Manual installation
- Using a requirements .txt file that defines the required packages and their version numbers
.
But before we start, let’s make sure pip is installed!
Python: Install
pip
First things first: we need to install pip itself. The good news is that Pip is probably already present in your system. Most Python installers also install Pip. The Python pip is already installed if you are using Python 2 >=2.7.9 or Python 3 >=3.4 downloaded from python.org. If you are working in a virtual environment, pip is also installed for you.
So, before you try to install Pip, make sure that it is not present on your system. Open a terminal (Linux/MacOS) or a Windows shell and type the following command:
pip help
If the pip command fails, try pip3 instead. Python 2 and 3 can be installed side-by-side on some systems. On those systems, pip is often installed under the name
pip3: pip3 help If that
didn’t work either, you can try the pip module that’s built into most modern Python installations:
python3 -m pip help
If that also failed, you need to install it yourself, so let’s take a look at how you can install it manually. You should do this only as a last resort, and I strongly suggest you first check if your Python installation went well first.
Install Pip on
Windows and Mac
On Windows and Mac, you can download a Python script to install pip, called get-pip.py. Download the file and run it with Python from a command prompt or terminal window:
python3 get-pip.py Make sure you are in the
directory where the script was downloaded
. Install pip on Linux (Ubuntu, Debian, Redhat) You can install pip with apt package manager on Debian,
Ubuntu, Linux
Mint and other Debian derivatives. It is the most recommended method and ensures that your system will remain in a consistent state.
$ sudo apt install python3-pip If
your system uses the yum package manager, you can try the following:
$ sudo yum install python-pip
Pip is part of EPEL (Extra Packages for Enterprise Linux), so you may need to enable it first.
If these methods fail, you can also download a Python script that will install pip for you, with the following commands
: $ curl “https://bootstrap.pypa.io/get-pip.py” -o “get-pip.py” …. $ python3 get-pip.py
pip Install Python
packages
I can’t stress this enough: preferably, you install packages within a virtual environment. And the good news: pip is present within your virtual environment by default. Because everything in our venv is installed locally, you don’t need to become a superuser with sudo or su and you don’t run the risk of package version conflicts.
That said, you can also install packages outside of Python venv. This is only recommended for more generic packages that you may need in many scripts, or if the package works as a more generic tool. Some example libraries that would fit this description would be Numpy and Pandas, a REPL alternative like ipython, or full environments like Jupyter Notebook.
Install locally (no
root or superuser) Fortunately,
these days installing Python on most operating systems configures your system in such a way that you don’t have to become an administrator (or root on a Unix system) to install packages outside of a venv
.
However, you will not always have superuser rights to install system-wide packages. For example, when working on a shared or locked system at work or school.
In such cases, you can install packages in the Python user installation directory for your platform using the -user option. This will normally mean that packages are installed somewhere on:
~/.local
- / on Unix-like systems
- APPDATA%localprogramspython on Windows
. %
In fact, installing packages in the local installation directory is usually the default these days when running outside of a virtual environment. So let’s try to update our account-wide pip installation first. Make sure you are not currently in a virtual environment and enter:
pip install -upgrade pip Depending on the
Python installer you have used and the system you are on, pip can try to install packages system-wide. As a result, if you don’t use something like sudo or become an administrator, you might get permission errors. If you get permission errors, it means that pip tried to install it system-wide. In that case, you can add the -user option to force its installation
in your system account: pip install -user -upgrade pip
These commands asked pip to install pip in your user account and update it if it is already installed. In my system, this gives me the message that the requirement is already satisfied, which means that pip is already updated.
Now, as an exercise, you can try to install ipython. It is a great alternative to the Python REPL standard.
Install Python packages in a venv
Now let’s try to install a package inside a virtual environment. For this example, I chose simplejson. First,
activate your virtual environment and then type: pip install simplejson
You have just installed a package within your virtual environment and as such it will only be accessible when you activate this venv
.
Install system-wide Python packages
Let me repeat first that I do not recommend this. If you jumped here without reading the rest of the topic, first read the previous section about installing packages in your user account or in a virtual environment.
On Linux, you can install a system-wide package by making it root. I strongly suggest you don’t, but here’s how to do it using sudo: sudo
pip install -upgrade pip pip installation requirements
.txt file
In a virtual environment, installing specific versions of packages is a good habit. It ensures that you get all the benefits of using virtual environments in the first place. After all, we do this to make sure our software always works as intended by setting specific dependency versions.
A requirements.txt file contains a simple list of dependencies, one per line. In its simplest form, it might look like this:
simplejson chardet
But what we really want is to fix the versions. That’s not difficult either
: chardet==3.0.4 simplejson==3.17.0 You can also relax these constraints a bit, using >= and <=,
or even a combination of them
: chardet>=3.0.0,<=3.1.0 simplejson>=3.17.0
You can use any of these specifiers: ==
, >, >=, <, <= Which range of
versions to choose
How do you know which range to use? It’s a topic that most tutorials and courses seem to avoid. Unfortunately, there are no strict rules for this. You will need to read the release notes and other package(s) in question.
For example, suppose you are using two packages, A and B. You may know that a certain feature was available in package A, version 3.1.0, so you need at least that version. But from the news, he learned that the author is planning a major revision of the Package A API in version 4.0.0. Also, package B is a bit stale and poorly maintained. It has been stuck on version 0.6.2 for years and requires version 3.1.6 or lower of package A (while A is currently at 3.5.0). You can define the following constraints to make sure everything works fine:
A>=3.1.0,<=3.1.6 B==0.6.2
You see, sometimes it takes a bit of juggling to define version constraints correctly. That’s why many developers use an alternative to Pip, such as Poetry or Pipenv. These tools offer advanced dependency management and will resolve all dependencies automatically, if possible. We take a close look at package managers later in this course. Right now, we’re making sure you understand the basics. This way, package managers will seem less magical and you’ll better understand what they’re doing for you. This is not the fun when it comes
to
freezing pip
Creating your requirements file using the pip freezing option can make your life a little easier. First, write your software and install all the requirements you need as you go. Once you’re done and everything seems to be working fine, use the following command:
$pip freeze > requirements.txt
Pip created a requirements.txt file with all currently installed dependencies, including version numbers. Neat! You can choose to relax the versions a bit, for example, to allow minor updates (bug fixes, security fixes) to a package. But remember that it’s also a risk: there’s always the possibility of ending up with a project that doesn’t work as intended because one of the requirements changed the behavior of your API or something.
Pip Install from a
file .txt requirements Finally, to install
all the dependencies listed in this file, use:
$ pip install -r requirements.txt
It’s an instruction you’ll see often when reading installation instructions for Python projects, and now you know exactly what it does and how it works!
Custom repository with pip install -i
The default PyPI repository is located in https://pypi.org/simple. However, you can also use an alternate repository. For example, if your company only allows a subset of approved packages from an internal mirror. Or maybe your company has a private mirror with its own package. This repository can be located on an HTTP URL or a file system location.
To specify a custom repository, use the -i or –
index-url option, like this:
pip install -i https://your-custom-repo/simple <package name> or pip install -i /path/to/your/custom-repo/simple <package name>
The URL must point to a PEP 503-compliant repository (the Simple Repository API) or to a local directory in the same format.
Editable installation with pip install -e
Pip has the option to perform an editable installation, which means you can install a package from a local source. But instead of copying the package files to some location on your system, pip creates symbolic links to the codebase you’re installing it from. This way, you can work on the project and make changes and be able to directly test those changes without constantly reinstalling new versions of the package.
There are several reasons why you may need this option:
- When you are creating your own
- you want to install
- so-called cutting-edge version of a package, directly from your source repository
package If you want to fix someone else’s package If
a
The editable
installation option is called with the -e option or the -editable option. Let’s see what pip has to say about this option: pip
install -help .. -e, -editable Install a project in editable mode (i.e., setuptools “development mode”) from a local project path or VCS URL. ..
Typically, you will work on a project using a version control system such as git. While inside the repository root, you would install the project with: pip install
-e .
If you are not in the root of the project, you can simply provide the path to the package source code instead of using the period.
Uninstalling
pip To uninstall a package with pip, we can use the
‘uninstall’ subcommand, for example
, to uninstall simplejson: pip uninstall simplejson
In line with the pip install command that uses a requirements file, you can also use that file to uninstall packages: pip uninstall requirements
-r.txt
More pip
commands We can also use pip to learn more about a package or currently installed packages. Pip also offers a search function. Let’s explore these additional commands.
Pip
list: list of installed packages
To list all currently installed packages using pip, use the following command: $ pip list
Package version – – async-generator 1.10 attrs 20.3.0 autopep8 1.5.7 …
It will display both package names and versions. When you run the command within a virtual environment, only venv packets are displayed. All account-wide or system-wide packages will be listed if you run it outside of a venv.
Pip show: get package details
Now that you know which packages are installed, you may need to inspect one in more detail. To do this, we have the subcommand
‘show’: $ pip show attrs Name: attrs Version: 20.3.0 Summary: Classes without repetitive Home: https://www.attrs.org/ Author: Hynek Schlawack Author-email: hs@ox.cx License: MIT Location: /usr/lib/python3/dist-packages Requires: Required by:
It’s a quick way to find up what a package is and does, and who created it.
Pip search: search for packages
We used to find all the packages that matched a certain chain using pip. Although convenient, this introduced a heavy load on PyPI’s servers. Unfortunately, they have decided to disable the feature. Luckily, we still have search engines like DuckDuckGo, Google, and Bing to find things!
The Python
Package
Index
We haven’t talked much about the Python package index yet, located at pypi.org. The site offers us a few things:
- You can create an account, which you only need when you want to publish your own packages.
- Offers a search function (more on this later)
- Shows us usage statistics, such as the total number
- We can browse by category
of packages and the user
To be honest, Python’s package index is a bit lacking, And I don’t think many people use the site itself.
PyPI search function
An example of how the site’s functionality doesn’t offer much value is the search function. If you know exactly what you’re looking for, that’s fine. For example, let’s try searching for the immensely popular application package. It will appear first and we can click on the information page here where we can see things like:
A description of the package The
- author’s name and email address
- link to the project’s home page, documentation, and source code
- list of historical
. A
releases
The authors of the package provide all this information, so keep that in mind.
Now suppose we can’t remember the name requests, and we look for the HTTP word instead
:
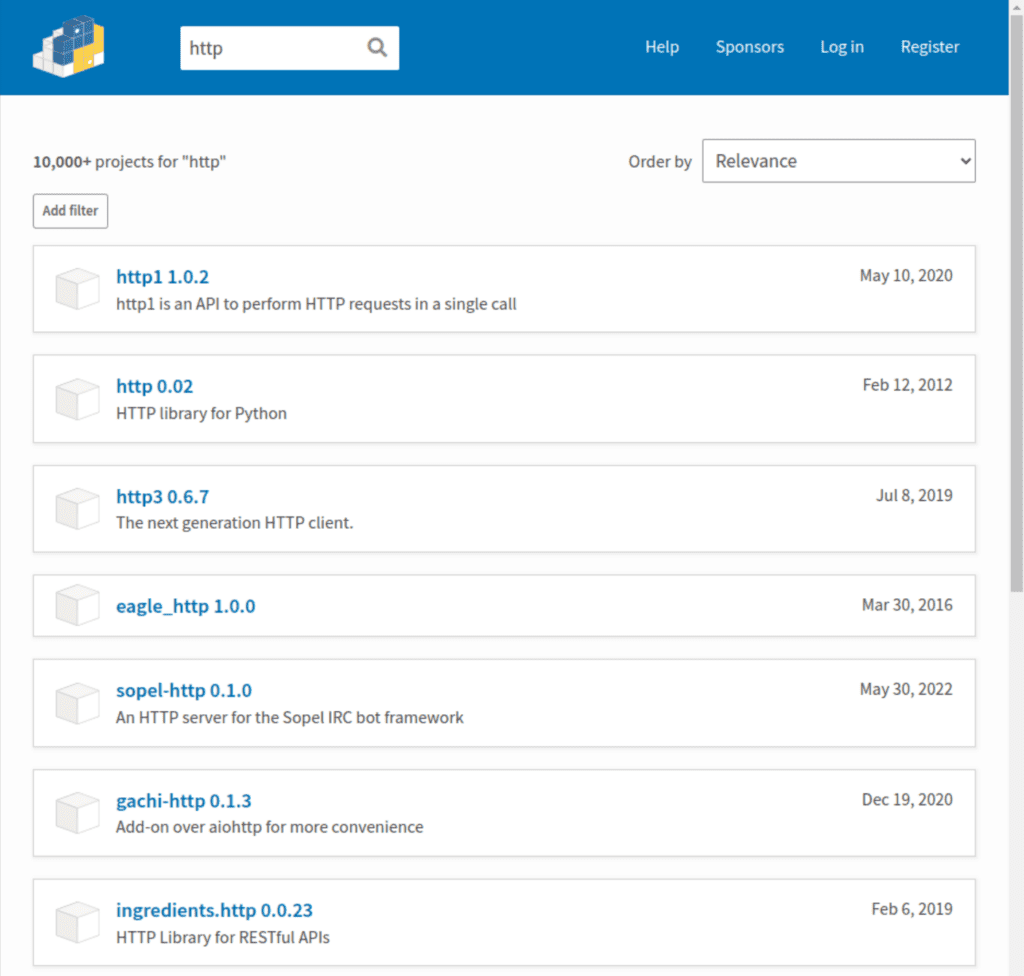
You can try and see for yourself if you want: the application package is nowhere to be found. So if you need to discover a library to do something specific, like make HTTP requests, I strongly suggest you use Google instead.
A simple Google search will yield that Python has a built-in HTTP library, which might be good enough. However, several of the early results also mention requests, which gives us a strong indicator that this package is used a lot.
PyPI Categories
If you browse through the categories, you will also find that they are not very useful. Fortunately, the Python community is strong, and many sites (like Python Land!) fill in the gaps and offer good advice on which packages to use.
So, in summary: the PyPI site will not be very useful for discovery. Still, it will be more than adequate to provide you with package information and links to documentation and source repositories.
Be careful what you install
It is extremely important to realize that anyone can upload packages to PyPI. This includes bad actors. Unfortunately, this is not a hypothetical problem. There have been several examples of malicious packages disguised as well-known projects. For example, someone could upload a package called requests2. There are many tricks that you and I would not think of to fool people. So this begs the question: how do you know which packets to trust and which to avoid?
How to know which packages are reliable
I will outline some steps you can take to greatly reduce the risk of installing a malicious package
.
- Do a Google search for the package name. Packages known as requests are mentioned a lot in articles, tutorials, and so on.
- Visit not only the PyPI page, but also the accompanying source code page (most often, it will be Github).
- Look for the package in libraries.io. They show some interesting metrics per package, one of which is called ‘Dependent Packages’. This number shows how many other packages use this package, giving you a strong indication of its popularity and reliability.
- Finally, when installing the package, check the name before pressing enter to avoid so-called typographic squatters.
Keep learning
This article is part of a free Python tutorial. You can navigate through the tutorial with the navigation buttons at the top and bottom of the article or use the navigation menu. Here are some other articles and links you can
check out: If you
- want a more feature-rich alternative, you should check out Poetry or Pipenv
- Check out my tutorial on virtual environments, if you haven’t already
- If you want to learn more about pip and all its options, head over to the official PyPI documentation.
- Read about pip configuration files
- The official section Python.org about editable installations (also called development mode)
.
.