Introduction
A bash function is a method used in shell scripts to group reusable blocks of code. This feature is available for most programming languages, known under different names, such as procedures, methods, or subroutines.
This article provides a complete description of bash functions, how they work, and how to use them.
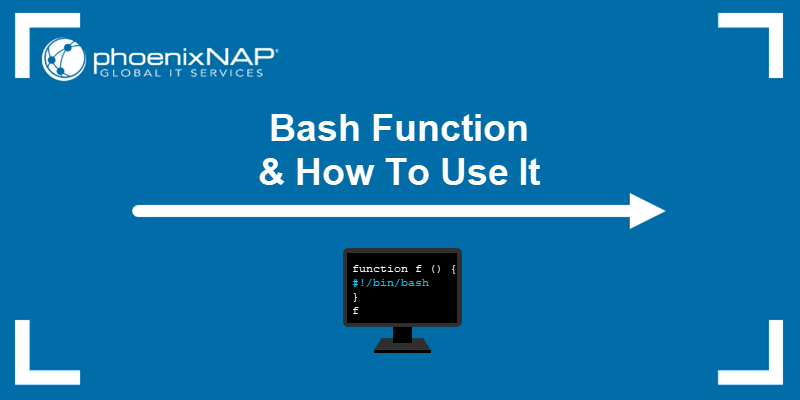
Prerequisites
A
- system running Linux
- Terminal access and Bash shell.
- A text editor for writing bash scripts (the guide uses Vim).
.
What are Bash functions?
A bash function is a technique for grouping reusable code snippets under a name for later use. The bash function is like a script within a script.

Using functions in bash scripting comes with two benefits:
1. A function is read directly from shell memory and stored for later use. Since computer memory is not an issue nowadays, using functions is faster than repeating code.
2. Functions help organize long shell scripts into modular and reusable code blocks. Pieces are easier to develop and maintain.
How to use Bash functions?
There are two ways to implement Bash functions:
- Within a shell script, where the definition of the function must be before any call
- Along with other bash alias commands and directly in the terminal as a command
to the function.
.
To use bash functions, follow the schemas below.
Bash function syntax
There are two
different ways to declare a bash function:
1. The most commonly used format is
: <function name> () { <commands> }
Alternatively, the same function can be a line: <function name
> () { <commands>; }
2. The alternative way to write a bash function is by using the reserved word
function: function <function name> { <commands> }
Or on one line:
function <function name> { <commands>; }
Take note of the following behaviors and tips when using functions:
- When typing on a line, commands must end with a semicolon (;), either in bash scripts or directly in the terminal
- Adding the function’s reserved word makes parentheses optional.
- Commands between the braces { <commands> } are called body of the function. The body can contain any number of statements, variables, loops, or conditional statements.
- Try to use descriptive names for functions. Although not required when testing functions and commands, friendly names help in configuration where other developers look at the code.
.
How to declare and call a function? A
function does not execute when it is declared. The body of the function is executed when it is invoked after the declaration. Follow the steps below to create a bash script with various syntax options:
1. Using your favorite text editor, create a shell script called syntax. If you are using Vim, run the following line in the terminal: vim
syntax.sh
2. Add the following code to the shell script:
# syntax.sh # Declaring functions using the reserved word function # Multiline function f1 { echo Hello I’m function 1 echo Goodbye! } # One-line function f2 { echo Hello I’m function 2; echo Bye!; } # Declaring functions without function reserved word # Multiline f3 () { echo Hello I’m function 3 echo Bye! } # One line f4 () { echo Hello I’m function 4; echo Bye!; } # Invoke functions f4 f3 f2 f1
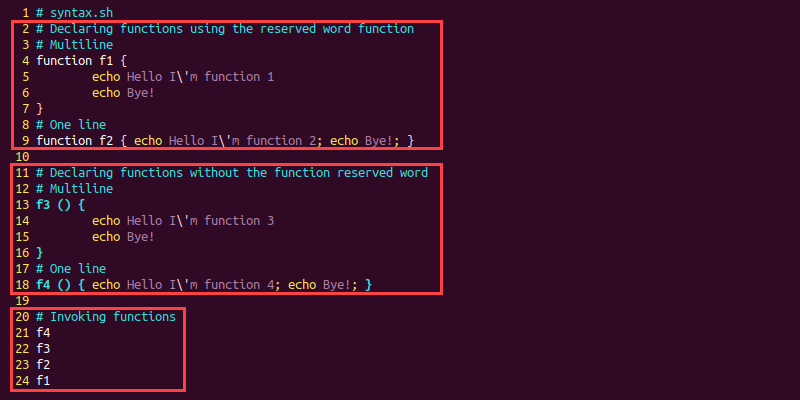
The script does the following:
- Lines 4-9 show how to define a function using the function’s reserved word. The f1 function uses a multiline syntax on lines 4-6, while f2 uses a line syntax on line 9.
- Lines 13-18 show the most familiar syntax. F3 is a multiline function defined on lines 13-16, while f4 on line 18 is the equivalent of a single line.
- Lines 21-24 invoke the previously defined functions and execute the commands in the bodies of the corresponding function. Calls are made in reverse order of definition.
3. Save the script and close Vim: :
wq
4. Make the syntax.sh file executable:
chmod +x syntax.sh
5. Finally, run the script to see
the output: ./syntax.sh
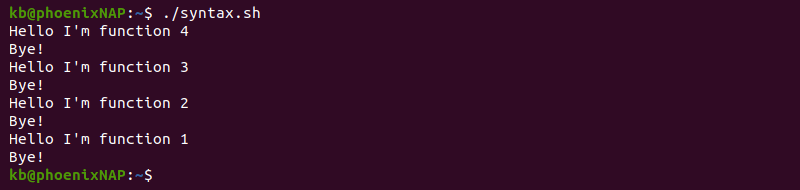
How to declare and call a function in the terminal?
To declare and use a function in the terminal:
1. Open the terminal and enter the following line:
my_function () { echo “Hello I’m a function”; echo “Bye!”; } 2. Execute the function
by entering
the function name in the terminal: my_function

The
output executes the commands in the function body
.
The function remains defined only in the current terminal session. To save for future sessions, add the code to the ~/.bashrc file.
Where is a Bash function defined?
To see where a bash function and its contents are defined, enter the following commands in the terminal:
1. Run the bash shell in debugger mode: bash -debugger
2. Check
the function source file with: declare -F <function name>
For example:
declare -F my_function
The output prints the function name, line number, and file location where the function definition is located
.
3. To
view the contents of the function, run: declare -f <function name>
For example:
declare -f
The debugging mode built into the declaration allows you to view the contents and location of the function without running the code.
How to remove a bash function?
If you need to free up a namespace occupied by
a function in the current terminal session, run: unset <function name>
For example:
unset my_function
The feature is no longer available in the current terminal session. However, if the code is in the ~/.bashrc file, everything is restored to normal in the next session.
Bash
function variables
Variables in bash are global by default and accessible from anywhere, including function bodies. Variables defined within a function are also global. Adding the local keyword makes the term accessible only within the function and child functions/processes.
At the dynamic scope, a local variable shades a global variable when the two are named after the two.
Try the following bash script to demonstrate how function variables work in bash:
1. Create a script named variable.sh:
vim variable.sh
2. Add the following code to the script
: var1=1 var2=1 change() { echo Inside the echo function Variable 1 is: $var 1 echo Variable 2 is: $var 2 local var1=5 var2=5 echo echo After the change within the echo function Variable 1 is locally $var 1 echo Variable 2 is globally $var 2 } echo Before invocation of the echo function Variable 1 is: $var 1 echo Variable 2 is: $var 2 echo change echo echo After function invocation echo Variable 1 is: $var 1 echo Variable 2 is: $var 2
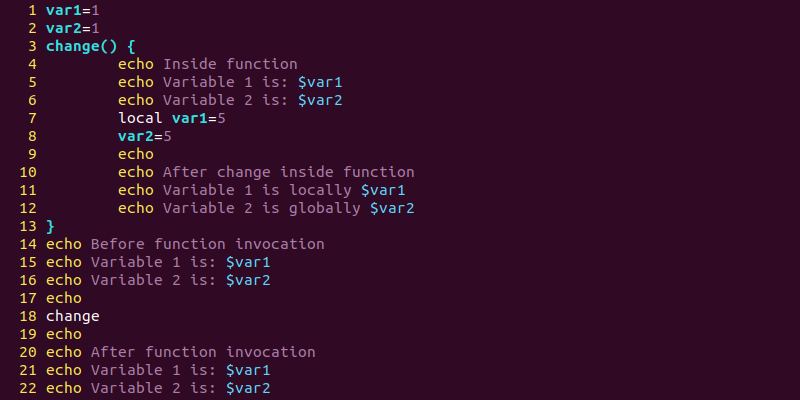
The script displays the following:
- Lines 1-2 declare the variables var1 and var2 and set them to 1.
- Lines 5-6 are inside the function body and print the variables to the console. Because the scope of the variable is global, the original values are printed.
- Line 7 declares a new local variable with the same name as the global variable var1. The local var1 hides the global var1 value because of the dynamic scope.
- Line 8 changes the value of the global variable var2.
- Lines 14-22 print variable values before and after the function is called.
3. Save the script and exit Vim: :
wq
4. Change the file permissions to executable:
chmod +x variable.sh
5. Run the script and analyze the results:
./variable.sh
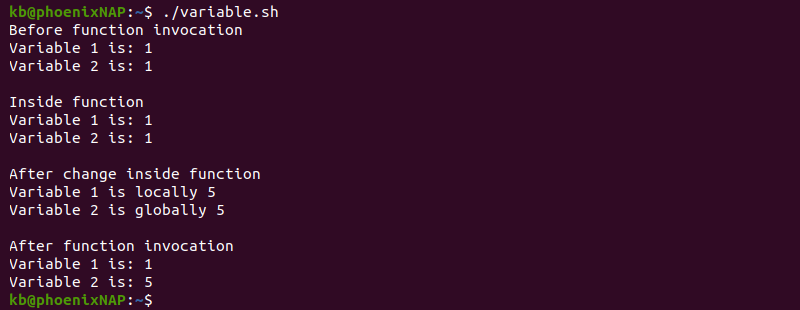
Variable values are printed to the console through changes made to the script
.
Bash
function arguments
To pass arguments to a function, add the parameters after the function call separated by spaces. The following table describes the options available when working with bash function arguments.
the
steps below to test how different arguments work in a function
.
1. Create a script called arguments:
vim arguments.sh
2. Add the following code
in the script: arguments() { echo The location of the function is $0 echo There are $# echo arguments “Argument 1 is $1” echo “Argument 2 is $2” echo “<$@>” and “<$*>” are equal. echo List the items in a for loop to see the difference! echo “* gives:” for arg in “$*”; echo “<$arg>”; done echo “@ gives:” for arg in “$@”; echo “<$arg>”; Done } arguments hello world
3. Save the script and exit Vim::
wq
4. Make the script executable:
chmod +x arguments.sh
5. Run the script:
./arguments.sh
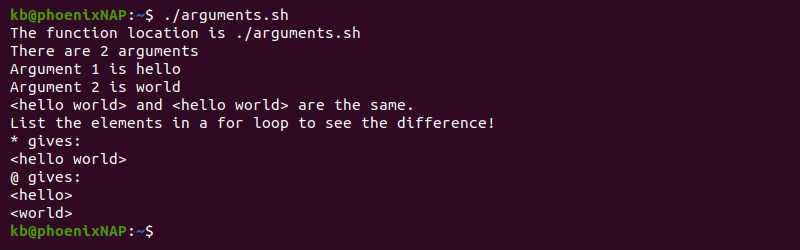
The output displays descriptive messages for each argument used
. Bash
Return
function
Bash functions differ from most programming languages when it comes to returning a value from a function. By default, bash returns the output state of the last command executed in the function body.
The following script shows how to specify the output state using return:
1. Create a script and name it
test.sh: vim test.sh
2. Add the following function to the file
: test_function() { echo Test return 100 } echo The output of the function is: test_function echo The output status is: echo $?
3. Save and close the text editor: :
wq
4. Change permissions:
chmod +x test.sh
5. Run the script to see
the output and output status of the function: ./test.sh
An alternative method is to echo the result of the function and assign the output to a variable. Edit the test.sh script with the following code:
test_function() { echo Test } result=$(test_function) echo $result is saved in a variable for later use
This method mimics how most programming languages work when using
functions.
Conclusion
After going through this tutorial, you should know how to use functions in bash scripting. Next, use our git command cheat sheet to help you automate repetitive git tasks using bash functions.